As a professional WordPress developer, I’ve implemented countless custom search solutions for clients across various industries. While WordPress comes with a basic search function out of the box, it often falls short for sites with complex content structures or specific search requirements.
In this expert guide, I’ll walk you through everything you need to know about creating powerful, tailored search experiences in WordPress—from understanding the native search limitations to implementing advanced custom solutions that will transform how users find content on your site.
Understanding WordPress Native Search and Its Limitations
Before diving into custom solutions, it’s important to understand how WordPress search works by default and why it might not meet your needs.
How WordPress Native Search Works
WordPress’s built-in search functionality is relatively straightforward:
- When a user submits a search query, WordPress creates a SQL query that looks for the search terms in post titles and content.
- By default, it searches only in the
posts
table, focusing on thepost_title
andpost_content
columns. - It returns results ordered by relevance (posts with the search term in the title rank higher than those with the term only in the content).
- The results are displayed using your theme’s
search.php
template (or falling back toindex.php
if no search template exists).
Common Limitations of Default WordPress Search
While functional for basic sites, the native search has several significant limitations:
- Limited Scope: Only searches post titles and content by default, ignoring custom fields, taxonomies, comments, and other content types.
- Poor Relevance Ranking: Basic matching algorithm that doesn’t account for term frequency, proximity, or importance.
- No Partial Word Matching: Doesn’t support prefix or suffix matching, so “develop” won’t find “development” or “developer.”
- No Typo Tolerance: No fuzzy matching or spelling correction capabilities.
- Performance Issues: Can become extremely slow on large sites due to inefficient database queries.
- No Content Weighting: Can’t prioritize newer content or specific post types without custom code.
- Limited Filter Options: No built-in way for users to refine search results by date, category, or other attributes.
For many WordPress sites, especially those with large content libraries or complex content structures like e-commerce WordPress sites, these limitations can significantly impact user experience and content discoverability.
Planning Your Custom Search Implementation
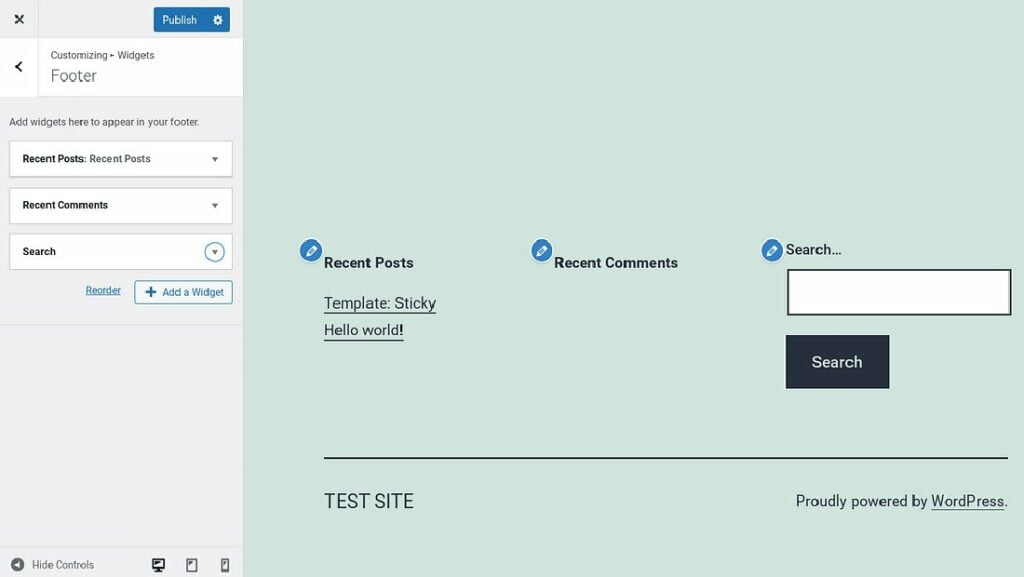
Before writing any code, it’s crucial to plan your search functionality based on your specific requirements.
Key Questions to Consider
- What content types need to be searchable? Posts, pages, custom post types, products, etc.
- What fields should be included in the search? Titles, content, excerpts, custom fields, taxonomies, etc.
- How should results be ranked? By relevance, date, popularity, or a combination?
- What search features do users need? Filters, autocomplete, instant results, faceted search, etc.
- What is your content volume? A few hundred posts vs. thousands of products requires different approaches.
- What are your performance requirements? How fast must search results load?
- What is your technical capacity? Custom code vs. plugin solutions.
Search Implementation Options
Based on your requirements, you have several implementation options:
- Enhance Native WordPress Search: Modify the default search query using WordPress hooks.
- Use a Search Plugin: Implement a dedicated search plugin like SearchWP, Relevanssi, or FacetWP.
- Integrate External Search Service: Connect to a third-party search service like Algolia or Elasticsearch.
- Build a Custom Search Solution: Create a completely custom search implementation from scratch.
Each approach has its advantages and considerations, which we’ll explore in detail.
Enhancing Native WordPress Search with Custom Code
For sites with moderate search requirements, enhancing the native WordPress search can be an efficient solution that doesn’t require external services or heavy plugins.
Expanding Search to Include Custom Post Types
By default, WordPress search only includes posts and pages. Here’s how to include custom post types:
function custom_search_post_types($query) {
// Only modify search queries on the frontend
if ($query->is_search() && !is_admin()) {
// Define which post types to include in search
$post_types = array('post', 'page', 'product', 'portfolio', 'team_member');
$query->set('post_type', $post_types);
}
return $query;
}
add_filter('pre_get_posts', 'custom_search_post_types');
This function hooks into the pre_get_posts
filter and modifies search queries to include additional post types. You would add your own custom post types to the array as needed.
Including Custom Fields in Search
To search within custom fields (meta data), you can use this approach:
function custom_search_include_meta($where, $wp_query) {
global $wpdb;
if (!$wp_query->is_search() || is_admin()) {
return $where;
}
$search_term = $wp_query->get('s');
// Skip if search term is empty
if (empty($search_term)) {
return $where;
}
// Define which meta keys to search
$meta_keys = array('_product_description', 'testimonial_author', 'project_details');
$meta_query = '';
foreach ($meta_keys as $key) {
$meta_query .= $wpdb->prepare(" OR (pm.meta_key = %s AND pm.meta_value LIKE %s)", $key, '%' . $wpdb->esc_like($search_term) . '%');
}
// Join posts table with postmeta table
$wp_query->query_vars['join'] .= " LEFT JOIN {$wpdb->postmeta} pm ON {$wpdb->posts}.ID = pm.post_id ";
// Group results to avoid duplicates
$wp_query->query_vars['groupby'] = "{$wpdb->posts}.ID";
// Add meta query to the WHERE clause
$where = preg_replace(
"/\(\s*{$wpdb->posts}.post_title\s+LIKE\s*(\'[^\']+\')\s*\)/",
"({$wpdb->posts}.post_title LIKE $1)" . $meta_query,
$where
);
return $where;
}
add_filter('posts_where', 'custom_search_include_meta', 10, 2);
This more complex function modifies the SQL query to include searches in specific custom fields.
Adding Taxonomy Terms to Search
To include category, tag, or custom taxonomy terms in search results:
function custom_search_include_taxonomies($where, $wp_query) {
global $wpdb;
if (!$wp_query->is_search() || is_admin()) {
return $where;
}
$search_term = $wp_query->get('s');
// Skip if search term is empty
if (empty($search_term)) {
return $where;
}
// Define which taxonomies to include
$taxonomies = array('category', 'post_tag', 'product_cat', 'expertise');
// Build array of taxonomy IDs
$taxonomy_ids = array();
foreach ($taxonomies as $taxonomy) {
$taxonomy_ids[] = $wpdb->prepare("%s", $taxonomy);
}
$tax_query = $wpdb->prepare(
" OR (
{$wpdb->posts}.ID IN (
SELECT object_id FROM {$wpdb->term_relationships}
WHERE term_taxonomy_id IN (
SELECT term_taxonomy_id FROM {$wpdb->term_taxonomy}
WHERE taxonomy IN (" . implode(',', $taxonomy_ids) . ")
AND term_id IN (
SELECT term_id FROM {$wpdb->terms}
WHERE name LIKE %s
)
)
)
)",
'%' . $wpdb->esc_like($search_term) . '%'
);
// Add taxonomy query to the WHERE clause
$where = preg_replace(
"/\(\s*{$wpdb->posts}.post_title\s+LIKE\s*(\'[^\']+\')\s*\)/",
"({$wpdb->posts}.post_title LIKE $1)" . $tax_query,
$where
);
return $where;
}
add_filter('posts_where', 'custom_search_include_taxonomies', 10, 2);
This allows users to find content by searching for category or tag names, which can be particularly useful for sites with well-structured taxonomies.
Improving Search Result Relevance
To improve the relevance of search results, you can modify how WordPress ranks search matches:
function custom_search_relevance($orderby, $query) {
global $wpdb;
if (!$query->is_search() || is_admin() || empty($query->get('s'))) {
return $orderby;
}
// Custom ordering that prioritizes title matches, then recent content
$orderby = "
CASE
WHEN {$wpdb->posts}.post_title LIKE '%{$wpdb->_real_escape($query->get('s'))}%' THEN 1
ELSE 2
END,
{$wpdb->posts}.post_date DESC
";
return $orderby;
}
add_filter('posts_orderby', 'custom_search_relevance', 10, 2);
This function creates a custom ordering that prioritizes title matches first, then sorts by publication date.
Creating a Better Search Form
The default WordPress search form is quite basic. Here’s how to create an enhanced version:
function custom_search_form($form) {
$form = '<form role="search" method="get" class="search-form" action="' . esc_url(home_url('/')) . '">
<div class="search-form-container">
<input type="search" class="search-field" placeholder="' . esc_attr_x('Search…', 'placeholder') . '" value="' . get_search_query() . '" name="s" />
<div class="search-filters">
<select name="post_type">
<option value="any">' . esc_html__('All Content', 'textdomain') . '</option>
<option value="post">' . esc_html__('Blog Posts', 'textdomain') . '</option>
<option value="page">' . esc_html__('Pages', 'textdomain') . '</option>
<option value="product">' . esc_html__('Products', 'textdomain') . '</option>
</select>
<select name="category_name">
<option value="">' . esc_html__('All Categories', 'textdomain') . '</option>';
// Get all categories
$categories = get_categories(array(
'orderby' => 'name',
'order' => 'ASC'
));
foreach ($categories as $category) {
$form .= '<option value="' . esc_attr($category->slug) . '">' . esc_html($category->name) . '</option>';
}
$form .= '</select>
</div>
<button type="submit" class="search-submit">' . esc_html__('Search', 'textdomain') . '</button>
</div>
</form>';
return $form;
}
add_filter('get_search_form', 'custom_search_form');
This creates a more advanced search form with filters for post type and category. You’ll need to add CSS to style it appropriately.
Implementing AJAX-Powered Live Search
One of the most user-friendly search enhancements is live search, which shows results as users type. Here’s how to implement it:
Step 1: Create the AJAX Handler
First, create a function to handle AJAX search requests:
function ajax_live_search() {
// Check for search query
$search_query = isset($_POST['query']) ? sanitize_text_field($_POST['query']) : '';
if (empty($search_query)) {
wp_send_json_error('No search term provided');
die();
}
// Define search arguments
$args = array(
'post_type' => array('post', 'page', 'product'),
'post_status' => 'publish',
's' => $search_query,
'posts_per_page' => 5,
);
// Run the query
$search = new WP_Query($args);
$results = array();
if ($search->have_posts()) {
while ($search->have_posts()) {
$search->the_post();
// Get featured image if available
$thumbnail = '';
if (has_post_thumbnail()) {
$thumbnail = get_the_post_thumbnail_url(get_the_ID(), 'thumbnail');
}
// Build result item
$results[] = array(
'title' => get_the_title(),
'link' => get_permalink(),
'thumbnail' => $thumbnail,
'post_type' => get_post_type(),
'excerpt' => wp_trim_words(get_the_excerpt(), 15, '...')
);
}
wp_reset_postdata();
}
wp_send_json_success($results);
die();
}
add_action('wp_ajax_live_search', 'ajax_live_search');
add_action('wp_ajax_nopriv_live_search', 'ajax_live_search');
Step 2: Add the JavaScript for Live Search
Next, add the JavaScript to handle the live search functionality:
function enqueue_live_search_scripts() {
wp_enqueue_script(
'live-search',
get_template_directory_uri() . '/js/live-search.js',
array('jquery'),
'1.0.0',
true
);
wp_localize_script(
'live-search',
'liveSearch',
array(
'ajaxurl' => admin_url('admin-ajax.php'),
'nonce' => wp_create_nonce('live_search_nonce'),
)
);
}
add_action('wp_enqueue_scripts', 'enqueue_live_search_scripts');
Step 3: Create the JavaScript File
Create a file called live-search.js
in your theme’s js directory:
jQuery(document).ready(function($) {
var searchTimer;
var searchInput = $('.search-field');
var resultsContainer = $('<div class="live-search-results"></div>');
// Add results container after search input
searchInput.after(resultsContainer);
// Hide results when clicking outside
$(document).on('click', function(e) {
if (!$(e.target).closest('.search-form-container').length) {
resultsContainer.hide();
}
});
// Process search input
searchInput.on('keyup', function() {
var query = $(this).val();
// Clear previous timer
clearTimeout(searchTimer);
// Hide results if query is empty
if (query.length < 3) {
resultsContainer.hide();
return;
}
// Set timer to prevent too many requests
searchTimer = setTimeout(function() {
$.ajax({
url: liveSearch.ajaxurl,
type: 'post',
data: {
action: 'live_search',
query: query,
nonce: liveSearch.nonce
},
beforeSend: function() {
resultsContainer.html('<div class="loading">Searching...</div>');
resultsContainer.show();
},
success: function(response) {
if (response.success && response.data.length > 0) {
resultsContainer.empty();
// Build results HTML
$.each(response.data, function(index, item) {
var resultItem = $('<div class="result-item"></div>');
if (item.thumbnail) {
resultItem.append('<div class="result-thumbnail"><img src="' + item.thumbnail + '" alt=""></div>');
}
var resultContent = $('<div class="result-content"></div>');
resultContent.append('<h4><a href="' + item.link + '">' + item.title + '</a></h4>');
resultContent.append('<span class="result-type">' + item.post_type + '</span>');
resultContent.append('<p>' + item.excerpt + '</p>');
resultItem.append(resultContent);
resultsContainer.append(resultItem);
});
// Add view all results link
resultsContainer.append('<div class="view-all"><a href="/?s=' + query + '">View all results</a></div>');
} else {
resultsContainer.html('<div class="no-results">No results found</div>');
}
},
error: function() {
resultsContainer.html('<div class="error">Error loading results</div>');
}
});
}, 500);
});
});
Step 4: Add CSS Styles
Add these styles to your theme’s stylesheet:
.search-form-container {
position: relative;
max-width: 600px;
margin: 0 auto;
}
.live-search-results {
position: absolute;
top: 100%;
left: 0;
right: 0;
background: #fff;
border: 1px solid #ddd;
border-top: none;
box-shadow: 0 4px 6px rgba(0,0,0,0.1);
z-index: 1000;
display: none;
max-height: 400px;
overflow-y: auto;
}
.result-item {
display: flex;
padding: 15px;
border-bottom: 1px solid #eee;
}
.result-thumbnail {
flex: 0 0 60px;
margin-right: 15px;
}
.result-thumbnail img {
width: 60px;
height: 60px;
object-fit: cover;
}
.result-content {
flex: 1;
}
.result-content h4 {
margin: 0 0 5px;
font-size: 16px;
}
.result-type {
display: inline-block;
font-size: 12px;
background: #f0f0f0;
padding: 2px 6px;
border-radius: 3px;
margin-bottom: 5px;
text-transform: capitalize;
}
.result-content p {
margin: 5px 0 0;
font-size: 14px;
color: #666;
}
.loading, .no-results, .error {
padding: 15px;
text-align: center;
}
.view-all {
padding: 10px;
text-align: center;
background: #f9f9f9;
}
This implementation creates a responsive, user-friendly live search experience that shows results as users type, with thumbnails and excerpts for better context.
Creating Advanced Filters for Search Results
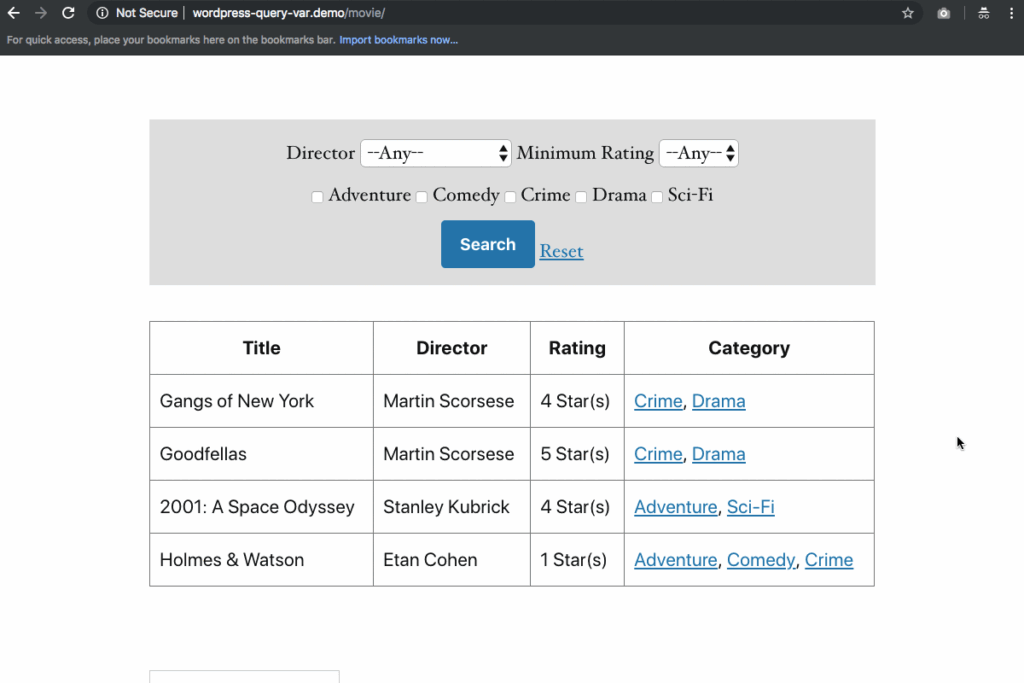
To give users more control over search results, you can implement advanced filtering options:
Step 1: Create a Custom Search Results Template
First, create a custom search.php
template in your theme:
<?php get_header(); ?>
<div class="search-results-container">
<header class="page-header">
<h1 class="page-title">
<?php printf(esc_html__('Search Results for: %s', 'textdomain'), '<span>' . get_search_query() . '</span>'); ?>
</h1>
<?php get_template_part('template-parts/search-filters'); ?>
</header>
<div class="search-results-content">
<?php if (have_posts()) : ?>
<div class="search-results-count">
<?php
global $wp_query;
printf(
esc_html(_n('%d result found', '%d results found', $wp_query->found_posts, 'textdomain')),
$wp_query->found_posts
);
?>
</div>
<div class="search-results-list">
<?php while (have_posts()) : the_post(); ?>
<article id="post-<?php the_ID(); ?>" <?php post_class('search-result-item'); ?>>
<?php if (has_post_thumbnail()) : ?>
<div class="search-result-thumbnail">
<a href="<?php the_permalink(); ?>">
<?php the_post_thumbnail('thumbnail'); ?>
</a>
</div>
<?php endif; ?>
<div class="search-result-content">
<header class="entry-header">
<?php the_title(sprintf('<h2 class="entry-title"><a href="%s" rel="bookmark">', esc_url(get_permalink())), '</a></h2>'); ?>
<div class="entry-meta">
<span class="post-type"><?php echo get_post_type_object(get_post_type())->labels->singular_name; ?></span>
<span class="post-date"><?php echo get_the_date(); ?></span>
<?php if (has_category()) : ?>
<span class="post-categories"><?php the_category(', '); ?></span>
<?php endif; ?>
</div>
</header>
<div class="entry-summary">
<?php the_excerpt(); ?>
</div>
<footer class="entry-footer">
<a href="<?php the_permalink(); ?>" class="read-more">
<?php esc_html_e('Read more', 'textdomain'); ?>
</a>
</footer>
</div>
</article>
<?php endwhile; ?>
</div>
<?php the_posts_pagination(); ?>
<?php else : ?>
<div class="no-results">
<h2><?php esc_html_e('No results found', 'textdomain'); ?></h2>
<p><?php esc_html_e('Sorry, but nothing matched your search terms. Please try again with different keywords or filters.', 'textdomain'); ?></p>
</div>
<?php endif; ?>
</div>
</div>
<?php get_sidebar(); ?>
<?php get_footer(); ?>
Step 2: Create the Search Filters Template Part
Create a file called template-parts/search-filters.php
:
<?php
// Get current search parameters
$current_query = get_search_query();
$current_post_type = isset($_GET['post_type']) ? sanitize_text_field($_GET['post_type']) : 'any';
$current_category = isset($_GET['category']) ? sanitize_text_field($_GET['category']) : '';
$current_date = isset($_GET['date_filter']) ? sanitize_text_field($_GET['date_filter']) : '';
$current_sort = isset($_GET['sort']) ? sanitize_text_field($_GET['sort']) : 'relevance';
?>
<div class="search-filters">
<form role="search" method="get" class="search-filter-form" action="<?php echo esc_url(home_url('/')); ?>">
<input type="hidden" name="s" value="<?php echo esc_attr($current_query); ?>" />
<div class="filter-row">
<div class="filter-group">
<label for="post-type-filter"><?php esc_html_e('Content Type', 'textdomain'); ?></label>
<select name="post_type" id="post-type-filter">
<option value="any" <?php selected($current_post_type, 'any'); ?>><?php esc_html_e('All Content', 'textdomain'); ?></option>
<option value="post" <?php selected($current_post_type, 'post'); ?>><?php esc_html_e('Blog Posts', 'textdomain'); ?></option>
<option value="page" <?php selected($current_post_type, 'page'); ?>><?php esc_html_e('Pages', 'textdomain'); ?></option>
<option value="product" <?php selected($current_post_type, 'product'); ?>><?php esc_html_e('Products', 'textdomain'); ?></option>
</select>
</div>
<div class="filter-group">
<label for="category-filter"><?php esc_html_e('Category', 'textdomain'); ?></label>
<select name="category" id="category-filter">
<option value="" <?php selected($current_category, ''); ?>><?php esc_html_e('All Categories', 'textdomain'); ?></option>
<?php
$categories = get_categories(array(
'orderby' => 'name',
'order' => 'ASC'
));
foreach ($categories as $category) {
printf(
'<option value="%s" %s>%s</option>',
esc_attr($category->slug),
selected($current_category, $category->slug, false),
esc_html($category->name)
);
}
?>
</select>
</div>
<div class="filter-group">
<label for="date-filter"><?php esc_html_e('Date', 'textdomain'); ?></label>
<select name="date_filter" id="date-filter">
<option value="" <?php selected($current_date, ''); ?>><?php esc_html_e('Any Time', 'textdomain'); ?></option>
<option value="day" <?php selected($current_date, 'day'); ?>><?php esc_html_e('Last 24 Hours', 'textdomain'); ?></option>
<option value="week" <?php selected($current_date, 'week'); ?>><?php esc_html_e('Last Week', 'textdomain'); ?></option>
<option value="month" <?php selected($current_date, 'month'); ?>><?php esc_html_e('Last Month', 'textdomain'); ?></option>
<option value="year" <?php selected($current_date, 'year'); ?>><?php esc_html_e('Last Year', 'textdomain'); ?></option>
</select>
</div>
<div class="filter-group">
<label for="sort-filter"><?php esc_html_e('Sort By', 'textdomain'); ?></label>
<select name="sort" id="sort-filter">
<option value="relevance" <?php selected($current_sort, 'relevance'); ?>><?php esc_html_e('Relevance', 'textdomain'); ?></option>
<option value="date" <?php selected($current_sort, 'date'); ?>><?php esc_html_e('Newest First', 'textdomain'); ?></option>
<option value="date_asc" <?php selected($current_sort, 'date_asc'); ?>><?php esc_html_e('Oldest First', 'textdomain'); ?></option>
<option value="title" <?php selected($current_sort, 'title'); ?>><?php esc_html_e('Title (A-Z)', 'textdomain'); ?></option>
<option value="title_desc" <?php selected($current_sort, 'title_desc'); ?>><?php esc_html_e('Title (Z-A)', 'textdomain'); ?></option>
</select>
</div>
</div>
<div class="filter-actions">
<button type="submit" class="filter-submit"><?php esc_html_e('Apply Filters', 'textdomain'); ?></button>
<a href="<?php echo esc_url(home_url('/?s=' . urlencode($current_query))); ?>" class="filter-reset"><?php esc_html_e('Reset Filters', 'textdomain'); ?></a>
</div>
</form>
</div>
Step 3: Modify the Search Query to Handle Filters
Add this function to process the filters:
function custom_search_filter_query($query) {
// Only modify search queries on the frontend
if (!$query->is_search() || is_admin() || !$query->is_main_query()) {
return;
}
// Post Type Filter
if (isset($_GET['post_type']) && $_GET['post_type'] !== 'any') {
$query->set('post_type', sanitize_text_field($_GET['post_type']));
} else {
// Default searchable post types
$query->set('post_type', array('post', 'page', 'product'));
}
// Category Filter
if (isset($_GET['category']) && !empty($_GET['category'])) {
$query->set('category_name', sanitize_text_field($_GET['category']));
}
// Date Filter
if (isset($_GET['date_filter']) && !empty($_GET['date_filter'])) {
$date_filter = sanitize_text_field($_GET['date_filter']);
$date_query = array();
switch ($date_filter) {
case 'day':
$date_query = array(
'after' => '1 day ago'
);
break;
case 'week':
$date_query = array(
'after' => '1 week ago'
);
break;
case 'month':
$date_query = array(
'after' => '1 month ago'
);
break;
case 'year':
$date_query = array(
'after' => '1 year ago'
);
break;
}
if (!empty($date_query)) {
$query->set('date_query', array($date_query));
}
}
// Sort Order
if (isset($_GET['sort']) && !empty($_GET['sort'])) {
$sort = sanitize_text_field($_GET['sort']);
switch ($sort) {
case 'date':
$query->set('orderby', 'date');
$query->set('order', 'DESC');
break;
case 'date_asc':
$query->set('orderby', 'date');
$query->set('order', 'ASC');
break;
case 'title':
$query->set('orderby', 'title');
$query->set('order', 'ASC');
break;
case 'title_desc':
$query->set('orderby', 'title');
$query->set('order', 'DESC');
break;
case 'relevance':
default:
// Default WordPress relevance sorting
break;
}
}
return $query;
}
add_action('pre_get_posts', 'custom_search_filter_query');
This implementation creates a robust search filtering system that allows users to refine results by content type, category, date, and sort order.
Implementing Search with Popular WordPress Plugins
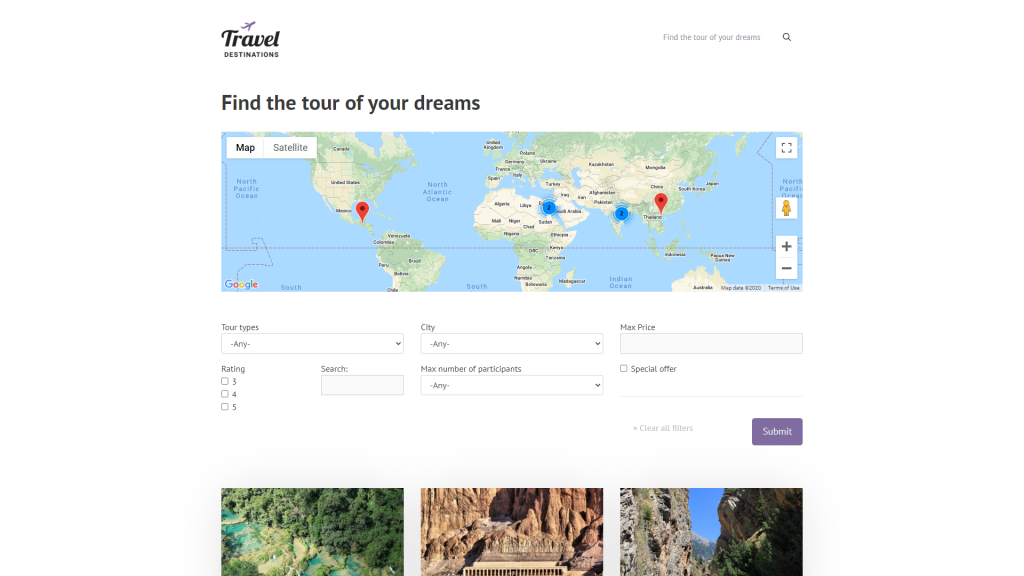
While custom code solutions offer maximum flexibility, there are several excellent plugins that can provide advanced search functionality with less development effort.
SearchWP: The Premium Search Solution
SearchWP is one of the most powerful search plugins available for WordPress, offering comprehensive indexing and search capabilities.
Key Features:
- Custom field and taxonomy integration
- PDF and document content indexing
- WooCommerce product search
- Custom weighting and relevance controls
- Related content suggestions
- Live Ajax search
Implementation Example:
After installing and activating SearchWP, you can customize its settings through the admin interface. For developers, here’s how to implement a custom search form with SearchWP:
function searchwp_custom_search_form() {
?>
<form role="search" method="get" class="searchwp-custom-form" action="<?php echo esc_url(home_url('/')); ?>">
<div class="search-input-wrapper">
<input type="search" name="s" value="<?php echo get_search_query(); ?>" placeholder="Search for..." />
<!-- Search engine selection (if you've created multiple engines) -->
<input type="hidden" name="engine" value="my_custom_engine" />
<!-- Post type filter -->
<select name="post_type">
<option value="any">All Content</option>
<option value="post">Blog Posts</option>
<option value="page">Pages</option>
<option value="product">Products</option>
</select>
<button type="submit">Search</button>
</div>
</form>
<?php
}
To programmatically use SearchWP in your theme or plugin:
function custom_searchwp_query($search_term, $args = array()) {
// Ensure SearchWP is active
if (!function_exists('SWP')) {
return array();
}
// Default arguments
$defaults = array(
'engine' => 'default',
'post_type' => array('post', 'page', 'product'),
'posts_per_page' => 10,
);
// Merge defaults with provided args
$args = wp_parse_args($args, $defaults);
// Run the search
$results = SWP()->search->run(array(
's' => $search_term,
'engine' => $args['engine'],
'post_type' => $args['post_type'],
'posts_per_page' => $args['posts_per_page'],
));
return $results;
}
Relevanssi: The Free Alternative
Relevanssi is a popular free alternative that significantly improves WordPress search functionality.
Key Features:
- Improved relevance algorithm
- Fuzzy matching for typo tolerance
- Partial word matching
- Custom field and taxonomy search
- Excerpt highlighting
- Search result weighting
Implementation Example:
After installing and activating Relevanssi, you can use the standard WordPress search form, as Relevanssi automatically hooks into the default search functionality. For custom implementation:
function relevanssi_custom_search($search_term, $args = array()) {
// Default arguments
$defaults = array(
'post_type' => array('post', 'page'),
'posts_per_page' => 10,
);
// Merge defaults with provided args
$args = wp_parse_args($args, $defaults);
// Add search term to args
$args['s'] = $search_term;
// Run the search query
$query = new WP_Query($args);
// Relevanssi automatically hooks into this query
return $query->posts;
}
To display highlighted excerpts in search results:
function display_relevanssi_excerpt() {
if (function_exists('relevanssi_the_excerpt')) {
relevanssi_the_excerpt();
} else {
the_excerpt();
}
}
FacetWP: For Faceted Search Interfaces
FacetWP specializes in creating faceted search interfaces, allowing users to filter search results by multiple criteria simultaneously.
Key Features:
- Drag-and-drop facet builder
- Real-time filtering without page reload
- Multiple facet types (checkboxes, dropdowns, sliders, etc.)
- WooCommerce integration
- URL history for shareable filtered results
- Template system for custom result layouts
Implementation Example:
After setting up FacetWP through its admin interface, you can display facets and results like this:
function display_facetwp_search() {
?>
<div class="search-container">
<div class="facet-sidebar">
<h3>Filter Results</h3>
<div class="facet-group">
<h4>Content Type</h4>
<?php echo facetwp_display('facet', 'post_type'); ?>
</div>
<div class="facet-group">
<h4>Categories</h4>
<?php echo facetwp_display('facet', 'category'); ?>
</div>
<div class="facet-group">
<h4>Publication Date</h4>
<?php echo facetwp_display('facet', 'date_range'); ?>
</div>
<button class="facetwp-reset" onclick="FWP.reset()">Reset Filters</button>
</div>
<div class="search-results">
<div class="facetwp-template">
<?php
// This loop will automatically update when facets are selected
if (have_posts()) {
while (have_posts()) {
the_post();
?>
<article class="search-result">
<h2><a href="<?php the_permalink(); ?>"><?php the_title(); ?></a></h2>
<div class="result-meta">
<?php echo get_post_type_object(get_post_type())->labels->singular_name; ?> |
<?php the_date(); ?>
</div>
<div class="result-excerpt">
<?php the_excerpt(); ?>
</div>
</article>
<?php
}
} else {
echo '<p>No results found.</p>';
}
?>
</div>
<div class="facetwp-pagination">
<?php echo facetwp_display('pager'); ?>
</div>
</div>
</div>
<?php
}
Integrating External Search Services
For large WordPress sites with extensive content, external search services can provide superior performance and features.
Elasticsearch Integration
Elasticsearch is a powerful, distributed search engine that can handle millions of documents with sub-second query times.
Implementation with ElasticPress Plugin:
ElasticPress is a free plugin that integrates WordPress with Elasticsearch:
// Check if ElasticPress is active and properly configured
function is_elasticpress_active() {
return class_exists('ElasticPress\Indexables') && ep_elasticsearch_alive();
}
// Custom search function using ElasticPress
function elastic_custom_search($search_term, $args = array()) {
if (!is_elasticpress_active()) {
// Fall back to default WordPress search if ElasticPress is not available
return new WP_Query(array_merge(array('s' => $search_term), $args));
}
// Default arguments
$defaults = array(
'post_type' => array('post', 'page'),
'posts_per_page' => 10,
);
// Merge defaults with provided args
$args = wp_parse_args($args, $defaults);
$args['s'] = $search_term;
// ElasticPress automatically integrates with WP_Query
$results = new WP_Query($args);
return $results;
}
Algolia Integration
Algolia is a hosted search service known for its lightning-fast results and advanced features like typo tolerance and geo-search.
Implementation with the Algolia Search Plugin:
After setting up the Algolia plugin and configuring your indices, you can implement a custom search interface:
function algolia_instant_search() {
?>
<div class="algolia-search-container">
<div id="search-input"></div>
<div class="algolia-search-results">
<div class="algolia-facets">
<div id="clear-refinements"></div>
<h3>Filter By</h3>
<div id="post-types-list"></div>
<div id="categories-list"></div>
<div id="tags-list"></div>
</div>
<div class="algolia-hits">
<div id="hits"></div>
<div id="pagination"></div>
</div>
</div>
</div>
<script src="https://cdn.jsdelivr.net/npm/instantsearch.js@4"></script>
<script>
document.addEventListener('DOMContentLoaded', function() {
const search = instantsearch({
indexName: 'wp_posts',
searchClient: algoliasearch(
'<?php echo esc_js(get_option('algolia_application_id')); ?>',
'<?php echo esc_js(get_option('algolia_search_api_key')); ?>'
)
});
// Initialize widgets
search.addWidgets([
instantsearch.widgets.searchBox({
container: '#search-input',
placeholder: 'Search for content...'
}),
instantsearch.widgets.hits({
container: '#hits',
templates: {
item: function(hit) {
return `
<div class="hit-item">
<h2><a href="${hit.permalink}">${hit._highlightResult.post_title.value}</a></h2>
<div class="hit-meta">
${hit.post_type_label} | ${new Date(hit.post_date).toLocaleDateString()}
</div>
<div class="hit-excerpt">
${hit._snippetResult.content.value}
</div>
</div>
`;
}
}
}),
instantsearch.widgets.pagination({
container: '#pagination'
}),
instantsearch.widgets.refinementList({
container: '#post-types-list',
attribute: 'post_type_label',
sortBy: ['name:asc'],
limit: 5,
showMore: true,
searchable: false,
operator: 'or',
templates: {
header: 'Content Types'
}
}),
instantsearch.widgets.refinementList({
container: '#categories-list',
attribute: 'taxonomies.category',
sortBy: ['name:asc'],
limit: 10,
showMore: true,
searchable: true,
operator: 'or',
templates: {
header: 'Categories'
}
}),
instantsearch.widgets.refinementList({
container: '#tags-list',
attribute: 'taxonomies.post_tag',
sortBy: ['name:asc'],
limit: 10,
showMore: true,
searchable: true,
operator: 'or',
templates: {
header: 'Tags'
}
}),
instantsearch.widgets.clearRefinements({
container: '#clear-refinements',
templates: {
resetLabel: 'Clear all filters',
}
})
]);
search.start();
});
</script>
<?php
}
Specialized Search Solutions for Different WordPress Sites
Different types of WordPress sites have unique search requirements. Here are specialized solutions for common site types:
E-commerce Product Search
For WordPress e-commerce sites, product search needs to handle attributes, variations, and pricing:
function woocommerce_custom_product_search($query) {
if (!is_admin() && $query->is_search() && $query->is_main_query() && isset($_GET['post_type']) && $_GET['post_type'] === 'product') {
// Set to search only products
$query->set('post_type', 'product');
// Include product variations
$query->set('post_status', array('publish'));
// Price filtering
if (isset($_GET['min_price']) && isset($_GET['max_price'])) {
$meta_query = $query->get('meta_query');
if (!is_array($meta_query)) {
$meta_query = array();
}
$meta_query[] = array(
'key' => '_price',
'value' => array(floatval($_GET['min_price']), floatval($_GET['max_price'])),
'type' => 'NUMERIC',
'compare' => 'BETWEEN'
);
$query->set('meta_query', $meta_query);
}
// Product attribute filtering
if (isset($_GET['product_attributes']) && is_array($_GET['product_attributes'])) {
$tax_query = $query->get('tax_query');
if (!is_array($tax_query)) {
$tax_query = array();
}
foreach ($_GET['product_attributes'] as $taxonomy => $terms) {
if (!empty($terms)) {
$tax_query[] = array(
'taxonomy' => sanitize_key($taxonomy),
'field' => 'slug',
'terms' => array_map('sanitize_title', (array) $terms),
'operator' => 'IN'
);
}
}
if (!empty($tax_query)) {
$tax_query['relation'] = 'AND';
$query->set('tax_query', $tax_query);
}
}
}
return $query;
}
add_action('pre_get_posts', 'woocommerce_custom_product_search');
Membership Site Search
For sites using WordPress membership plugins, you might need to restrict search results based on user access:
function membership_restricted_search($query) {
// Only modify search queries for non-admins
if (!is_admin() && $query->is_search() && $query->is_main_query() && !current_user_can('administrator')) {
// Get current user's membership level
$user_id = get_current_user_id();
$membership_level = get_user_meta($user_id, 'membership_level', true);
// Define which post IDs the user can access
$accessible_posts = array();
// If user is not logged in, show only public content
if ($user_id === 0) {
$args = array(
'post_type' => array('post', 'page'),
'posts_per_page' => -1,
'fields' => 'ids',
'meta_query' => array(
array(
'key' => 'content_access',
'value' => 'public',
'compare' => '='
)
)
);
$public_posts = get_posts($args);
$accessible_posts = array_merge($accessible_posts, $public_posts);
} else {
// For logged-in users, include content based on their membership level
$args = array(
'post_type' => array('post', 'page'),
'posts_per_page' => -1,
'fields' => 'ids',
'meta_query' => array(
'relation' => 'OR',
array(
'key' => 'content_access',
'value' => 'public',
'compare' => '='
),
array(
'key' => 'content_access',
'value' => $membership_level,
'compare' => '='
)
)
);
$member_posts = get_posts($args);
$accessible_posts = array_merge($accessible_posts, $member_posts);
}
// If no accessible posts, show no results
if (empty($accessible_posts)) {
$query->set('post__in', array(0)); // No posts have ID 0, so this returns no results
} else {
$query->set('post__in', $accessible_posts);
}
}
return $query;
}
add_action('pre_get_posts', 'membership_restricted_search');
Multilingual Site Search
For multilingual WordPress sites using plugins like WPML or Polylang:
function multilingual_search_results($query) {
if (!is_admin() && $query->is_search() && $query->is_main_query()) {
// Get current language
$current_lang = '';
// For WPML
if (function_exists('icl_object_id') && defined('ICL_LANGUAGE_CODE')) {
$current_lang = ICL_LANGUAGE_CODE;
}
// For Polylang
elseif (function_exists('pll_current_language')) {
$current_lang = pll_current_language();
}
if (!empty($current_lang)) {
// For WPML
if (function_exists('icl_object_id')) {
$query->set('suppress_filters', false); // WPML hooks into this
}
// For Polylang
elseif (function_exists('pll_current_language')) {
$query->set('lang', $current_lang);
}
}
}
return $query;
}
add_action('pre_get_posts', 'multilingual_search_results');
For sites using WordPress translation plugins, this ensures search results match the user’s current language.
Performance Optimization for WordPress Search
Custom search implementations can impact site performance if not properly optimized. Here are key techniques to ensure your search functionality remains fast:
Database Query Optimization
function optimize_search_query($query) {
if (!is_admin() && $query->is_search() && $query->is_main_query()) {
// Limit fields being retrieved for better performance
$query->set('fields', 'ids'); // Get only post IDs initially
// Use a custom callback to get the necessary data
add_filter('the_posts', function($posts, $q) {
if (!$q->is_main_query() || empty($posts)) {
return $posts;
}
// Get only the fields we need
global $wpdb;
$post_ids = implode(',', array_map('intval', $posts));
$custom_posts = $wpdb->get_results(
"SELECT ID, post_title, post_excerpt, post_date, post_type
FROM {$wpdb->posts}
WHERE ID IN ({$post_ids})
ORDER BY FIELD(ID, {$post_ids})"
);
// Convert to proper post objects if needed
foreach ($custom_posts as $post) {
$post->post_status = 'publish';
$post->post_content = ''; // Don't load full content for search results
$post->comment_count = 0; // Skip comment counting
}
return $custom_posts;
}, 10, 2);
}
return $query;
}
add_action('pre_get_posts', 'optimize_search_query');
Implementing Search Caching
function cached_search_results($query) {
if (!is_admin() && $query->is_search() && $query->is_main_query()) {
$search_term = get_search_query();
$cache_key = 'search_' . md5($search_term . serialize($query->query_vars));
// Try to get cached results
$cached_results = get_transient($cache_key);
if ($cached_results !== false) {
// Return cached results and skip the main query
$query->posts = $cached_results['posts'];
$query->post_count = count($query->posts);
$query->found_posts = $cached_results['found_posts'];
$query->max_num_pages = $cached_results['max_num_pages'];
// Short-circuit the main query
add_filter('posts_pre_query', function($posts, $q) use ($query, $cached_results) {
if ($q === $query) {
return $cached_results['posts'];
}
return $posts;
}, 10, 2);
} else {
// Cache the results after the query is complete
add_filter('the_posts', function($posts, $q) use ($cache_key, $query) {
if ($q->is_main_query() && $q->is_search()) {
set_transient($cache_key, array(
'posts' => $posts,
'found_posts' => $query->found_posts,
'max_num_pages' => $query->max_num_pages
), HOUR_IN_SECONDS); // Cache for 1 hour
}
return $posts;
}, 10, 2);
}
}
return $query;
}
add_action('pre_get_posts', 'cached_search_results');
This caching implementation significantly improves performance for repeated searches, which are common on most sites.
Implementing Search Results Pagination
For large result sets, proper pagination is essential:
function custom_search_pagination($query) {
if (!is_admin() && $query->is_search() && $query->is_main_query()) {
// Set posts per page
$query->set('posts_per_page', 10);
// Handle custom pagination parameters
if (isset($_GET['results_page'])) {
$page = intval($_GET['results_page']);
$query->set('paged', $page);
}
}
return $query;
}
add_action('pre_get_posts', 'custom_search_pagination');
// Display custom pagination
function display_custom_search_pagination() {
global $wp_query;
if (!$wp_query->is_search()) {
return;
}
$current_page = max(1, get_query_var('paged'));
$total_pages = $wp_query->max_num_pages;
if ($total_pages <= 1) {
return;
}
echo '<div class="custom-pagination">';
// Previous page link
if ($current_page > 1) {
echo '<a href="' . add_query_arg('results_page', $current_page - 1) . '" class="prev-page">« Previous</a>';
}
// Page numbers
$range = 2; // How many pages to show on each side of current page
for ($i = 1; $i <= $total_pages; $i++) {
if ($i == 1 || $i == $total_pages || ($i >= $current_page - $range && $i <= $current_page + $range)) {
if ($i == $current_page) {
echo '<span class="current-page">' . $i . '</span>';
} else {
echo '<a href="' . add_query_arg('results_page', $i) . '" class="page-number">' . $i . '</a>';
}
} elseif ($i == $current_page - $range - 1 || $i == $current_page + $range + 1) {
echo '<span class="dots">...</span>';
}
}
// Next page link
if ($current_page < $total_pages) {
echo '<a href="' . add_query_arg('results_page', $current_page + 1) . '" class="next-page">Next »</a>';
}
echo '</div>';
}
Conclusion: Choosing the Right Search Solution for Your WordPress Site
WordPress search functionality can range from simple to highly sophisticated, depending on your site’s needs and resources. Here’s a summary of the approaches we’ve covered:
- Enhancing Native WordPress Search: Ideal for small to medium sites with basic search requirements. It requires custom code but doesn’t add plugin overhead.
- AJAX-Powered Live Search: Provides a modern, user-friendly search experience with real-time results. Best for sites where search is a key user interaction point.
- Advanced Filters for Search Results: Helps users narrow down results, particularly valuable for sites with diverse content types or large content libraries.
- Search Plugins: Solutions like SearchWP, Relevanssi, and FacetWP offer advanced features with minimal development effort. Best for sites needing sophisticated search without custom development.
- External Search Services: Algolia and Elasticsearch provide enterprise-level search capabilities for large sites with thousands of content items or high traffic.
When deciding which approach to take, consider:
- Content Volume: How much content needs to be searchable?
- User Expectations: What search experience do your users expect?
- Technical Resources: Do you have development capabilities for custom solutions?
- Budget: Can you invest in premium plugins or external services?
- Performance Requirements: How fast must search results load?
For most WordPress sites, a combination of approaches often works best – perhaps enhancing the native search with custom code for better relevance, while implementing AJAX for a smoother user experience.
Remember that search functionality directly impacts user experience and content discoverability. A well-implemented search solution can significantly improve engagement metrics and help users find exactly what they’re looking for on your site.
If you need help implementing custom search functionality for your WordPress site, consider working with a WordPress expert who specializes in performance optimization and custom development.
By following the techniques and best practices outlined in this guide, you can create a powerful, efficient search experience that perfectly matches your site’s unique requirements—without relying solely on plugins or external services.